Selection API
The Selection API allows apps to read and replace the content of the user's current selection. This enables powerful features like image effects and text manipulation.
Our design guidelines help you create a high-quality app that easily passes app review.
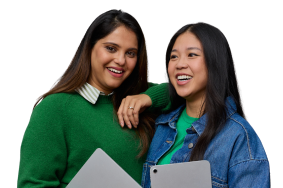
Supported content types
Apps can read and replace the following types of content:
- Images (not including SVGs)
- Plaintext
- Richtext
- Videos
Be aware that different design ingredients may contain the same types of content. For example, both image elements and page backgrounds contain image content that can be read by an app.
In the future, we intend to support more types of content.
Permissions
The Selection API requires different permissions depending on how your app uses the API:
- If your app only reads content, enable
canva:design:content:read
. - If your app replaces plaintext or richtext content, enable:
canva:design:content:read
canva:design:content:write
- If your app replaces image or video content, enable:
canva:design:content:read
canva:design:content:write
canva:asset:private:read
canva:asset:private:write
The Apps SDK will throw an error if the required permissions are not enabled.
To learn more, see Configuring permissions.
Listening for selection events
When a user selects one or more pieces of content, Canva emits a selection event. This event contains information about the selected content.
To listen for selection events:
-
Import the
useSelection
hook from theutils
directory:import { useSelection } from "utils/use_selection_hook";TS -
Call the hook, passing in the type of content to detect the selection of:
const selectedContent = useSelection("plaintext");TSThe following values are supported:
"image"
"plaintext"
"richtext"
"video"
The hook runs:
- when a user selects one or more pieces of content
- immediately, if a piece of content is already selected
The result of calling the hook is the selection event:
console.log(selectedContent);
Checking if content is selected
The selection event contains a count
property that contains the number of selected content items. When content isn't selected, this value is 0
. You can use this behavior to detect if content is selected:
const isContentSelected = selectedContent.count > 0;
This is useful for updating the user interface in response to the user's selection, such as by only enabling a button when content is selected:
<Button variant="primary" disabled={!isContentSelected}>Click me</Button>
Reading selected content
The selection event has a read
method that returns an object with a contents
property. This property contains an array of objects. Each object represents an individual piece of content, such as the image used for a page background. The available properties in this object depends on the type of content.
Images
If the selection event contains image content, each object contains an asset reference that points to an asset in Canva's backend:
const draft = await selectedContent.read();for (const content of draft.contents) {console.log(content.ref);}
You can use the getTemporaryUrl
method to get the URL of the underlying image file:
import { getTemporaryUrl } from "@canva/asset";const { url } = await getTemporaryUrl({type: "image",ref: content.ref,});console.log(url);
Keep in mind that:
- The URL always points to the full-size version of the image, even if the image is cropped or exists in a frame(opens in a new tab or window). This is the intended behavior as the user can always change the crop or frame.
- The returned URL is temporary and expires after a short period of time. Your app should immediately download the image to ensure that it has ongoing access to it.
Plaintext
If the selection event contains plaintext content, each object contains the plaintext:
const draft = await selectedContent.read();for (const content of draft.contents) {console.log(content.text);}
All formatting is stripped out, but the text may contain line breaks in the form \n
.
Richtext
If the selection event contains richtext content, each object is a richtext range that provides methods for interacting with that particular portion of richtext:
const draft = await selectedContent.read();for (const content of draft.contents) {// Get the text with formatting informationconst regions = content.readTextRegions();for (const region of regions) {// The plaintext content of a regionconsole.log(region.text);// The formatting information of a regionconsole.log(region.formatting);}}
To learn more, see Creating text.
Videos
If the selection event contains video content, each object contains an asset reference that points to an asset in Canva's backend:
const draft = await selectedContent.read();for (const content of draft.contents) {console.log(content.ref);}
You can use the getTemporaryUrl
method to get the URL of the underlying video file:
import { getTemporaryUrl } from "@canva/asset";const { url } = await getTemporaryUrl({type: "video",ref: content.ref,});console.log(url);
Keep in mind that:
- The URL always points to the full-size version of the video, even if the video is cropped or exists in a frame(opens in a new tab or window). This is the intended behavior as the user can always change the crop or frame.
- The returned URL is temporary and expires after a short period of time. Your app should immediately download the video to ensure that it has ongoing access to it.
Replacing selected content
In addition to reading selected content, apps can replace selected content.
The basic workflow for replacing content is always the same, regardless of the content type:
-
Read the current selection:
const draft = await selectedContent.read();TS -
Loop through the contents and transform them:
for (const content of draft.contents) {// Content-specific transformation logic goes here}TSEach
content
item is an object. The properties in this object depend on the type of content. -
Save the changes:
await draft.save();TSChanges made to
contents
won't be reflected in the user's design until the changes are saved.
Images
For images, the transformation process within the loop involves:
- Downloading the image using
getTemporaryUrl
- Transforming the image data
- Uploading the transformed image as an asset
- Replacing the
ref
of the asset - Setting a
parentRef
property to theref
of the original asset (see Deriving assets(opens in a new tab or window))
For example:
import { getTemporaryUrl, upload } from "@canva/asset";const draft = await selectedContent.read();for (const content of draft.contents) {// Get the image URLconst { url } = await getTemporaryUrl({type: "image",ref: content.ref,});// Transform the image (implementation varies)const transformedImage = await transformImage(url);// Upload the transformed imageconst asset = await upload({type: "image",url: transformedImage.url,mimeType: transformedImage.mimeType,thumbnailUrl: transformedImage.thumbnailUrl,parentRef: content.ref,aiDisclosure: "none",});// Replace the image refcontent.ref = asset.ref;}await draft.save();
You can transform images either on the frontend or backend:
To transform images via the app's frontend:
- Download each image
- Draw each image into an
HTMLCanvasElement
- Apply transformations
- Get the data URL(opens in a new tab or window)
The following code sample contains a reusable function that handles this logic:
import { getTemporaryUrl, ImageMimeType, ImageRef } from "@canva/asset";/*** Downloads and transforms a raster image.* @param ref - A unique identifier that points to an image asset in Canva's backend.* @param transformer - A function that transforms the image.* @returns The data URL and MIME type of the transformed image.*/async function transformRasterImage(ref: ImageRef,transformer: (ctx: CanvasRenderingContext2D, imageData: ImageData) => void): Promise<{ dataUrl: string; mimeType: ImageMimeType }> {// Get a temporary URL for the assetconst { url } = await getTemporaryUrl({type: "image",ref,});// Download the imageconst response = await fetch(url, { mode: "cors" });const imageBlob = await response.blob();// Extract MIME type from the downloaded imageconst mimeType = imageBlob.type;// Warning: This doesn't attempt to handle SVG imagesif (!isSupportedMimeType(mimeType)) {throw new Error(`Unsupported mime type: ${mimeType}`);}// Create an object URL for the imageconst objectURL = URL.createObjectURL(imageBlob);// Define an image element and load image from the object URLconst image = new Image();image.crossOrigin = "Anonymous";await new Promise((resolve, reject) => {image.onload = resolve;image.onerror = () => reject(new Error("Image could not be loaded"));image.src = objectURL;});// Create a canvas and draw the image onto itconst canvas = document.createElement("canvas");canvas.width = image.width;canvas.height = image.height;const ctx = canvas.getContext("2d");if (!ctx) {throw new Error("CanvasRenderingContext2D is not available");}ctx.drawImage(image, 0, 0);// Get the image data from the canvas to manipulate pixelsconst imageData = ctx.getImageData(0, 0, canvas.width, canvas.height);transformer(ctx, imageData);// Put the transformed image data back onto the canvasctx.putImageData(imageData, 0, 0);// Clean up: Revoke the object URL to free up memoryURL.revokeObjectURL(objectURL);// Convert the canvas content to a data URL with the original MIME typeconst dataUrl = canvas.toDataURL(mimeType);return { dataUrl, mimeType };}function isSupportedMimeType(input: string): input is "image/jpeg" | "image/heic" | "image/png" | "image/webp" {// This does not include "image/svg+xml"const mimeTypes = ["image/jpeg", "image/heic", "image/png", "image/webp"];return mimeTypes.includes(input);}
To use the transformRasterImage
, function, pass an image reference in as the first argument and a function for transforming the image as the second argument. The following usage inverts the colors of an image:
const { dataUrl, mimeType } = await transformRasterImage(content.ref,(_, { data }) => {// Invert the colors of each pixelfor (let i = 0; i < data.length; i += 4) {data[i] = 255 - data[i];data[i + 1] = 255 - data[i + 1];data[i + 2] = 255 - data[i + 2];}});console.log("The data URL of the transformed image is:", dataUrl);
When processing images in the frontend, the final image is uploaded to Canva's backend from the user's device. This approach may incur data charges for users, especially on mobile.
To transform images via your app's backend, use the Fetch API to send the URL of the image to the app's backend:
const response = await fetch("http://localhost:3000/invert-image", {method: "POST",headers: {"Content-Type": "application/json",},body: JSON.stringify({url,}),});
Your app's backend must verify incoming HTTP requests. To learn more, see HTTP request verification.
On the backend, transform the image and return a URL that Canva can use to download the new image:
import axios from "axios";import cors from "cors";import express from "express";import Jimp from "jimp";import path from "path";// TODO: Add the URL of the server here — it must be available to Canva's backendconst PUBLIC_SERVER_URL = "<INSERT_PUBLIC_SERVER_URL_HERE>";const app = express();app.use(cors());app.use(express.json());app.use("/uploads", express.static(path.join(__dirname, "uploads")));app.post("/invert-image", async (req, res) => {// Download the imageconst response = await axios({url: req.body.url,method: "get",responseType: "arraybuffer",});// Invert the image's colorsconst image = await Jimp.read(Buffer.from(response.data));image.invert();// Save the transformed image to "uploads" directoryconst id = Date.now().toString();const imageName = `${id}.jpg`;const imagePath = path.join(__dirname, "uploads", imageName);await image.writeAsync(imagePath);// Create a thumbnail of the transformed imageconst thumbnailName = `${id}_thumbnail.jpg`;const thumbnailPath = path.join(__dirname, "uploads", thumbnailName);const thumbnailWidth = 300;const thumbnailHeight = Jimp.AUTO;image.resize(thumbnailWidth, thumbnailHeight);await image.writeAsync(thumbnailPath);// Get the image's MIME typeconst mimeType = image.getMIME();// Return the URLs of the transformed image and thumbnailres.json({id,url: `${PUBLIC_SERVER_URL}/uploads/${imageName}`,thumbnailUrl: `${PUBLIC_SERVER_URL}/uploads/${thumbnailName}`,mimeType,});});app.listen(process.env.PORT || 3000, () => {console.log("The server is running...");});
On the frontend, parse the response to access the returned data:
// Parse the response as JSONconst json = await response.json();console.log(json.url); // => "https://..."
The URL of the new image must be available via the public internet. This is because Canva's backend must be able to download the image. To learn more, see Assets.
Plaintext
For plaintext, modify the text
property of each content item:
const draft = await selectedContent.read();for (const content of draft.contents) {// Transform the text however you needcontent.text = `${content.text} was modified!`;}await draft.save();
Richtext
For richtext, each content
object is a richtext range that exposes methods for reading and modifying formatted text. Here's an example that creates a rainbow effect:
const draft = await selectedContent.read();// Keep track of the current color across text regionslet currentColorIndex = 0;for (const content of draft.contents) {// Get the text regionsconst regions = content.readTextRegions();// Loop through each text regionfor (const region of regions) {// Loop through each character in the regions's textfor (let i = 0; i < region.text.length; i++) {// Get the color for the current characterconst colorIndex = (currentColorIndex + i) % RAINBOW_COLORS.length;const color = RAINBOW_COLORS[colorIndex];// Format the current charactercontent.formatText({ start: region.offset + i, length: 1 }, { color });}// Update the current colorcurrentColorIndex += region.text.length;}}await draft.save();
To learn more about richtext manipulation, see Richtext ranges.
Videos
For videos, the process is similar to images:
- Downloading the video using
getTemporaryUrl
- Transforming the video data
- Uploading the transformed video as an asset
- Replacing the
ref
of the asset - Setting a
parentRef
property to theref
of the original asset (see Deriving assets(opens in a new tab or window))
For example:
import { getTemporaryUrl, upload } from "@canva/asset";const draft = await selectedContent.read();for (const content of draft.contents) {// Get the video URLconst { url } = await getTemporaryUrl({type: "video",ref: content.ref,});// Transform the video (typically on backend)const transformedVideo = await transformVideo(url);// Upload the transformed videoconst asset = await upload({type: "video",url: transformedVideo.url,mimeType: transformedVideo.mimeType,thumbnailImageUrl: transformedVideo.thumbnailImageUrl,thumbnailVideoUrl: transformedVideo.thumbnailVideoUrl,parentRef: content.ref,aiDisclosure: "none",});// Replace the video refcontent.ref = asset.ref;}await draft.save();
Video transformation typically happens on the backend due to processing requirements:
// Frontend: Send video URL to backendconst response = await fetch("http://localhost:3000/transform-video", {method: "post",headers: {"Content-Type": "application/json",},body: JSON.stringify({url,}),});// Parse the responseconst json = await response.json();
Your app's backend must verify incoming HTTP requests. To learn more, see HTTP request verification.
The transformed video URL must be publicly accessible. To learn more, see Assets.
Known limitations
- Apps can only read and replace raster images — not vector images.
- You can't replace one type of content with a different type of content.
- If multiple pieces of content are selected, the ordering of the content is not stable and should not be relied upon.
API reference
Code samples
Images
import React from "react";import { Button, Rows } from "@canva/app-ui-kit";import { getTemporaryUrl, upload, ImageMimeType, ImageRef } from "@canva/asset";import { useSelection } from "utils/use_selection_hook";import * as styles from "styles/components.css";export function App() {const selectedContent = useSelection("image");const isContentSelected = selectedContent.count > 0;async function handleClick() {if (!isContentSelected) {return;}const draft = await selectedContent.read();for (const content of draft.contents) {// Download and transform the imageconst newImage = await transformRasterImage(content.ref,(_, { data }) => {for (let i = 0; i < data.length; i += 4) {data[i] = 255 - data[i];data[i + 1] = 255 - data[i + 1];data[i + 2] = 255 - data[i + 2];}});// Upload the transformed imageconst asset = await upload({type: "image",url: newImage.dataUrl,mimeType: newImage.mimeType,thumbnailUrl: newImage.dataUrl,parentRef: content.ref,aiDisclosure: "none",});// Replace the imagecontent.ref = asset.ref;}await draft.save();}return (<div className={styles.scrollContainer}><Rows spacing="2u"><Buttonvariant="primary"disabled={!isContentSelected}onClick={handleClick}>Replace selected image content</Button></Rows></div>);}/*** Downloads and transforms a raster image.* @param ref - A unique identifier that points to an image asset in Canva's backend.* @param transformer - A function that transforms the image.* @returns The data URL and MIME type of the transformed image.*/async function transformRasterImage(ref: ImageRef,transformer: (ctx: CanvasRenderingContext2D, imageData: ImageData) => void): Promise<{ dataUrl: string; mimeType: ImageMimeType }> {// Get a temporary URL for the assetconst { url } = await getTemporaryUrl({type: "image",ref,});// Download the imageconst response = await fetch(url, { mode: "cors" });const imageBlob = await response.blob();// Extract MIME type from the downloaded imageconst mimeType = imageBlob.type;// Warning: This doesn't attempt to handle SVG imagesif (!isSupportedMimeType(mimeType)) {throw new Error(`Unsupported mime type: ${mimeType}`);}// Create an object URL for the imageconst objectURL = URL.createObjectURL(imageBlob);// Define an image element and load image from the object URLconst image = new Image();image.crossOrigin = "Anonymous";await new Promise((resolve, reject) => {image.onload = resolve;image.onerror = () => reject(new Error("Image could not be loaded"));image.src = objectURL;});// Create a canvas and draw the image onto itconst canvas = document.createElement("canvas");canvas.width = image.width;canvas.height = image.height;const ctx = canvas.getContext("2d");if (!ctx) {throw new Error("CanvasRenderingContext2D is not available");}ctx.drawImage(image, 0, 0);// Get the image data from the canvas to manipulate pixelsconst imageData = ctx.getImageData(0, 0, canvas.width, canvas.height);transformer(ctx, imageData);// Put the transformed image data back onto the canvasctx.putImageData(imageData, 0, 0);// Clean up: Revoke the object URL to free up memoryURL.revokeObjectURL(objectURL);// Convert the canvas content to a data URL with the original MIME typeconst dataUrl = canvas.toDataURL(mimeType);return { dataUrl, mimeType };}function isSupportedMimeType(input: string): input is "image/jpeg" | "image/heic" | "image/png" | "image/webp" {// This does not include "image/svg+xml"const mimeTypes = ["image/jpeg", "image/heic", "image/png", "image/webp"];return mimeTypes.includes(input);}
Plaintext
import React from "react";import { Button, Rows } from "@canva/app-ui-kit";import { useSelection } from "utils/use_selection_hook";import * as styles from "styles/components.css";export function App() {const selectedContent = useSelection("plaintext");const isContentSelected = selectedContent.count > 0;async function handleClick() {if (!isContentSelected) {return;}const draft = await selectedContent.read();for (const content of draft.contents) {content.text = `${content.text} was modified!`;}await draft.save();}return (<div className={styles.scrollContainer}><Rows spacing="2u"><Buttonvariant="primary"disabled={!isContentSelected}onClick={handleClick}>Replace selected plaintext content</Button></Rows></div>);}
Richtext
import React from "react";import { Button, Rows } from "@canva/app-ui-kit";import { useSelection } from "utils/use_selection_hook";import * as styles from "styles/components.css";const RAINBOW_COLORS = ["#FF0000","#FF7F00","#FFFF00","#00FF00","#0000FF","#4B0082","#8B00FF",];export function App() {const selectedContent = useSelection("richtext");const isContentSelected = selectedContent.count > 0;async function handleClick() {if (!isContentSelected) {return;}// Get a snapshot of the currently selected textconst draft = await selectedContent.read();// Keep track of the current color across text regionslet currentColorIndex = 0;// Loop through all selected richtext contentfor (const content of draft.contents) {// Get the text regionsconst regions = content.readTextRegions();// Loop through each text regionfor (const region of regions) {// Loop through each character in the regions's textfor (let i = 0; i < region.text.length; i++) {// Get the color for the current characterconst colorIndex = (currentColorIndex + i) % RAINBOW_COLORS.length;const color = RAINBOW_COLORS[colorIndex];// Format the current charactercontent.formatText({ start: region.offset + i, length: 1 },{ color });}// Update the current colorcurrentColorIndex += region.text.length;}}// Commit the changesawait draft.save();}return (<div className={styles.scrollContainer}><Rows spacing="2u"><Buttonvariant="primary"disabled={!isContentSelected}onClick={handleClick}>Replace selected richtext content</Button></Rows></div>);}
Videos
import React from "react";import { Button, Rows } from "@canva/app-ui-kit";import { useSelection } from "utils/use_selection_hook";import { upload } from "@canva/asset";import * as styles from "styles/components.css";export function App() {const selectedContent = useSelection("video");const isContentSelected = selectedContent.count > 0;async function handleClick() {if (!isContentSelected) {return;}const draft = await selectedContent.read();for (const content of draft.contents) {// Upload the replacement videoconst asset = await upload({type: "video",mimeType: "video/mp4",url: "https://www.canva.dev/example-assets/video-import/beach-video.mp4",thumbnailImageUrl:"https://www.canva.dev/example-assets/video-import/beach-thumbnail-image.jpg",thumbnailVideoUrl:"https://www.canva.dev/example-assets/video-import/beach-thumbnail-video.mp4",parentRef: content.ref,aiDisclosure: "none",});// Replace the videocontent.ref = asset.ref;}await draft.save();}return (<div className={styles.scrollContainer}><Rows spacing="2u"><Buttonvariant="primary"disabled={!isContentSelected}onClick={handleClick}>Replace selected video content</Button></Rows></div>);}